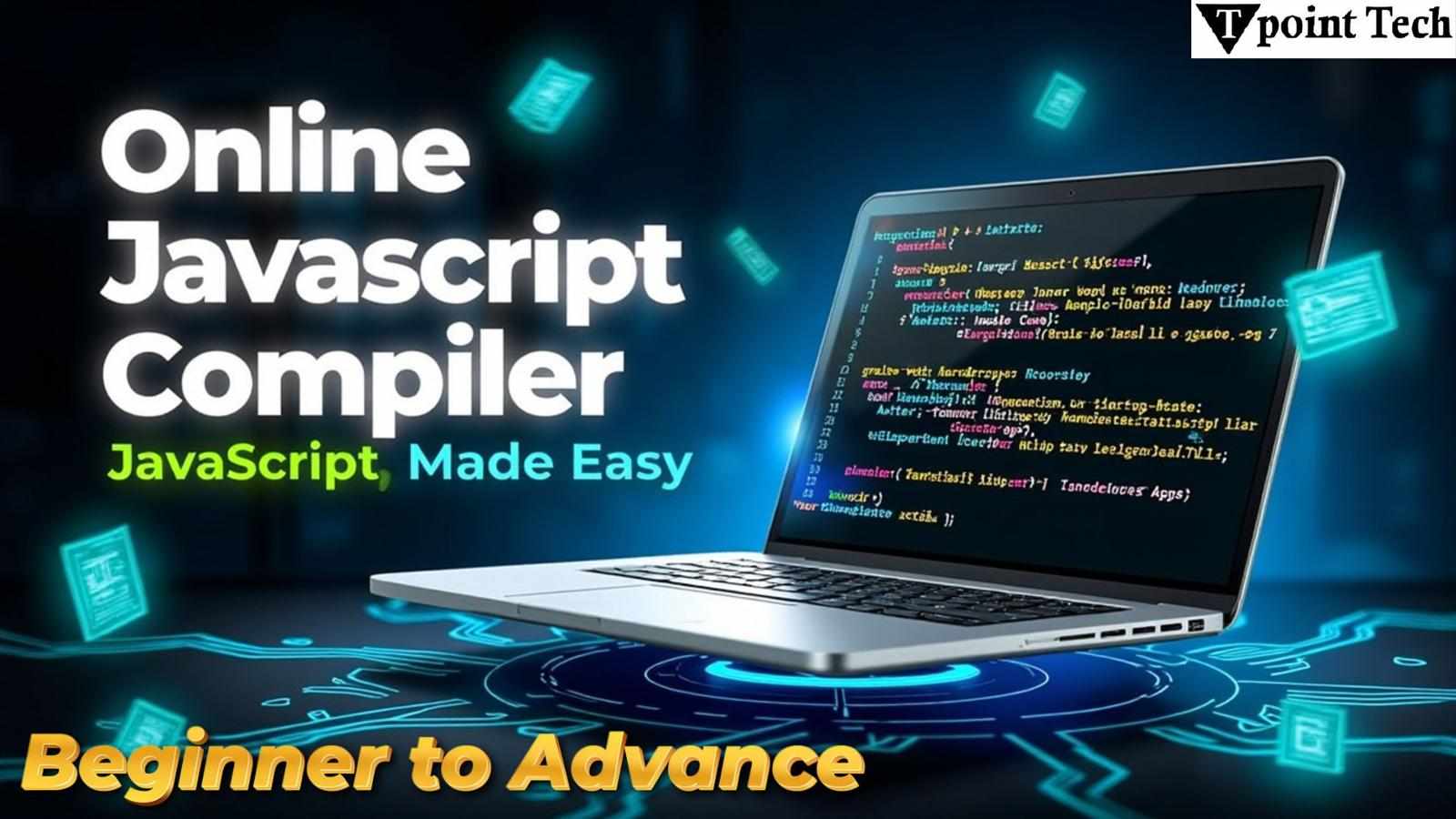
JavaScript is one of the most widely-used programming languages today. It’s the cornerstone of web development and allows you to create dynamic and interactive web pages. For beginners eager to dive into JavaScript, starting out can seem a bit overwhelming. However, with the help of an online JavaScript compiler, learning and testing JavaScript has never been easier.
In this guide, we will explore JavaScript basics and demonstrate how an online JavaScript compiler can help you experiment with the language without needing to set up a development environment on your computer.
Why JavaScript?
JavaScript plays a critical role in web development by enabling the creation of interactive websites. While HTML provides structure and CSS handles styling, JavaScript adds functionality to web pages, such as forms, animations, and even real-time updates.
Here are a few reasons why learning JavaScript is essential:
Ubiquity: JavaScript runs in every modern web browser, making it a universal tool for web development.
Versatility: From creating simple animations to building complex web applications, JavaScript is incredibly versatile.
Community and Resources: The JavaScript community is vast, and you’ll have access to countless tutorials, libraries, and frameworks to enhance your skills.
What is an Online JavaScript Compiler?
An online JavaScript compiler is a web-based tool that allows you to write and run JavaScript code directly from your browser. These tools are especially useful for beginners because they eliminate the need for complex setups or installations. You can test and debug code in real-time, which is a great way to see immediate results and learn from your mistakes.
With an online JavaScript compiler, all you need is an internet connection and a browser. You don’t need to worry about configuring your development environment, making these compilers the perfect starting point for beginners.
Benefits of Using an Online JavaScript Compiler
Instant Access: The moment you open the website of an online JavaScript compiler, you’re ready to start coding. There’s no need to install anything on your computer.
No Setup Required: These tools are pre-configured and ready to run JavaScript code immediately, saving you the headache of configuring your development environment.
Interactive Learning: With an online JavaScript compiler, you can test your code instantly and get real-time feedback. This interactive process is key to learning programming effectively.
Cross-Platform: Since everything happens in your browser, you can access your work from virtually any device, whether you’re on a laptop, tablet, or smartphone.
Collaboration: Some online compilers allow you to share your code with others, making it easier to collaborate with peers or instructors. You can even integrate your code with version control systems like GitHub for better code management.
How to Start Using an Online JavaScript Compiler
Step 1: Choose a Compiler
There are several online JavaScript compilers available. Popular options include:
JSFiddle: A collaborative platform that allows you to write JavaScript alongside HTML and CSS.
CodePen: Great for building interactive user interfaces and testing small JavaScript snippets.
Replit: A simple online compiler that lets you run JavaScript, as well as many other programming languages.
JSBin: Allows you to write and test JavaScript, HTML, and CSS in real time.
Simply choose one of these platforms to begin writing your JavaScript code.
Step 2: Write Your Code
Once you’re on the compiler’s website, you’ll typically see a split screen layout with areas to write JavaScript, HTML, and CSS. You can start by writing a basic JavaScript program. For example, try a simple "Hello, World!" program:
javascript
CopyEdit
console.log("Hello, World!");
This code simply outputs the text "Hello, World!" to the console. It’s a great first step in familiarizing yourself with JavaScript syntax.
Step 3: Run Your Code
After writing your code, most online JavaScript compilers have a "Run" button. Simply click it, and the results will be displayed. In this case, you’ll see "Hello, World!" in the console area.
Step 4: Experiment and Learn
One of the best ways to learn JavaScript is by experimenting. Try modifying your code, adding new functions, or changing the logic. For example, try creating a function that returns the sum of two numbers:
javascript
CopyEdit
function add(a, b) {
return a + b;
}
console.log(add(3, 5)); // Outputs: 8
By seeing immediate feedback from the online JavaScript compiler, you can understand how different code changes impact your program.
Tips for Learning JavaScript Effectively
Understand the Basics First: Before diving into more complex JavaScript concepts, make sure you understand the fundamentals like variables, functions, and loops.
Practice Regularly: Coding is a skill that improves with practice. Spend time each day writing small snippets of JavaScript to reinforce what you’ve learned.
Use Resources: Utilize online tutorials, forums, and guides to deepen your understanding. Platforms like MDN Web Docs, W3Schools, and freeCodeCamp are great places to start.
Experiment in the Browser Console: Many online JavaScript compilers also allow you to work directly in your browser’s developer tools. Experimenting in this console is a great way to try out new ideas and quickly see results.
Conclusion
JavaScript is an incredibly powerful language, and mastering it will open up a world of opportunities in web development. An online JavaScript compiler makes it easier than ever for beginners to dive in, practice, and experiment with code in a hassle-free environment. By regularly using these tools, you can steadily build your knowledge and become proficient in JavaScript.
Whether you’re writing your first lines of code or developing a complex web application, an online JavaScript compiler is the perfect companion for any programmer. So, start coding today and unlock the power of JavaScript right from your browser!
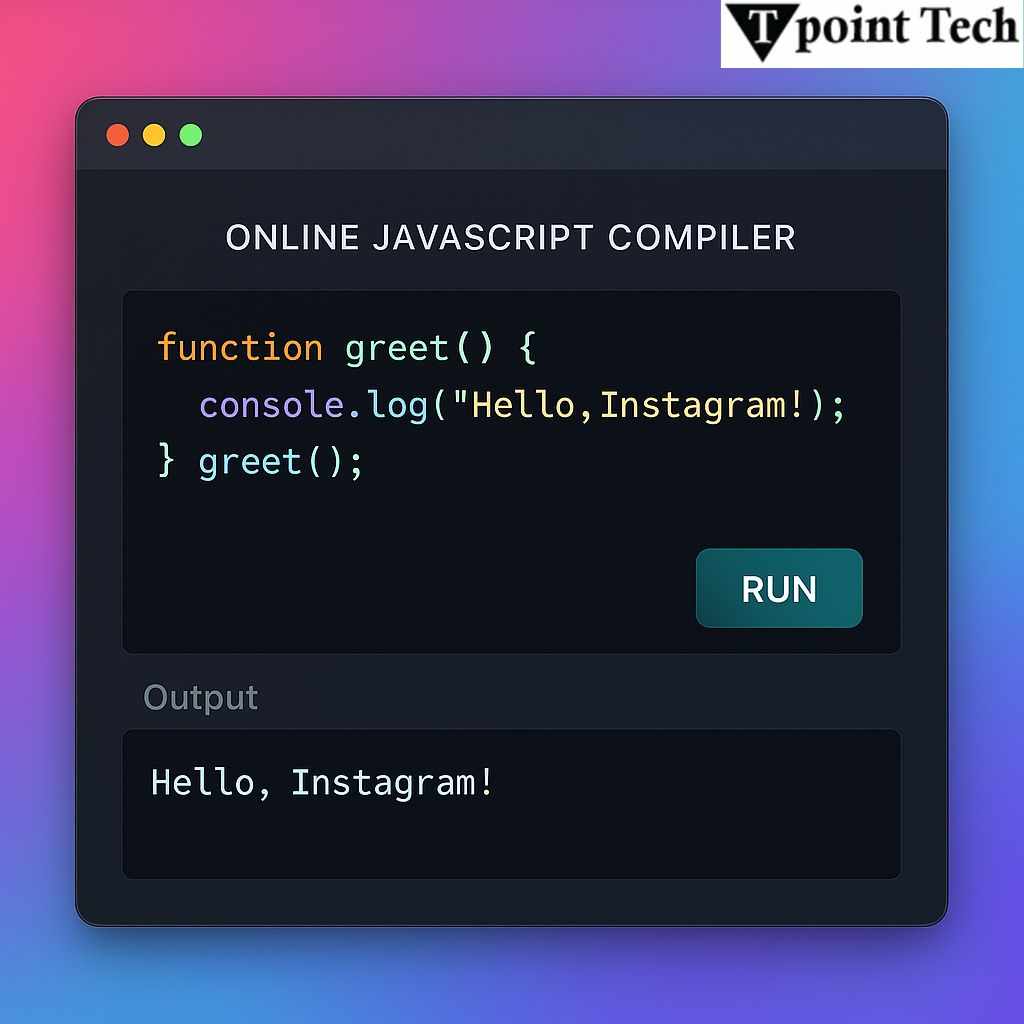
Write a comment ...